일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 | 31 |
- ViewModel
- DataBinding
- notification
- HTTP
- 코틀린
- CustomView
- 알고리즘
- Behavior
- recyclerview
- View
- Android
- onMeasure
- Coroutine
- CoordinatorLayout
- hilt
- Navigation
- Algorithm
- 알림
- room
- CollapsingToolbarLayout
- activity
- lifecycle
- sqlite
- LiveData
- onLayout
- 안드로이드
- AppBarLayout
- 백준
- BOJ
- kotlin
- Today
- Total
개발일지
Android in A..Z - HTTP (Retrofit2) 본문
Retrofit2
Android HTTP 통신에는 URLConnection, Volley Retrofi2 등이 존재합니다. Retrofit2는 동기/비동기 등 다양한 기능을 지원하고 Annotation을 통해 코드가 간결하다는 장점이 있습니다.
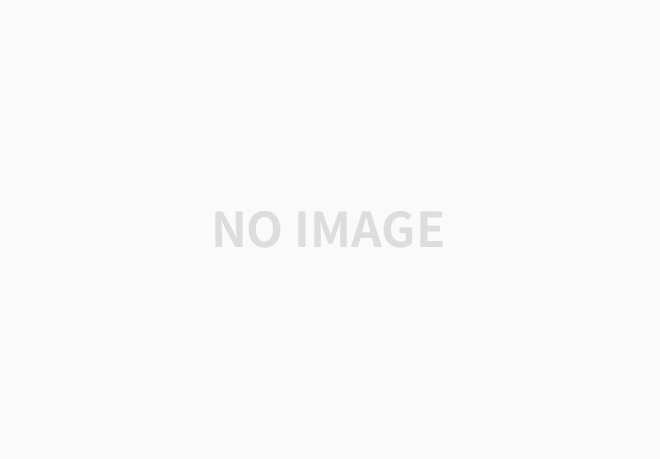

Dependency
gson : Response를 Google의 Gson을 통해 DTO로 매핑시켜줄 때 사용한다.
scalars : String형식으로 Response를 받을 때 사용한다.
dependencies {
// Retrofit
implementation 'com.squareup.retrofit2:converter-gson:2.6.2'
implementation 'com.squareup.retrofit2:converter-scalars:2.6.2'
implementation 'com.squareup.retrofit2:retrofit:2.6.2'
}
RetrofitBuilder
object로 선언하여 어디서든 쉽게 접근할 수 있게 만들었습니다.
object RetrofitBuilder {
val retrofit: RetrofitHTTP = Retrofit.Builder()
.baseUrl("${Server.PROTOCOL}://${Server.IP}:${Server.PORT}")
.addConverterFactory(ScalarsConverterFactory.create())
.addConverterFactory(GsonConverterFactory.create())
.build()
.create(RetrofitHTTP::class.java)
interface RetrofitHTTP {
@Headers(
"Content-Type: application/x-www-form-urlencoded"
)
@GET("/application/x-www-form-urlencoded")
fun getUrlencoded(@Query("parameter1") parameter1: Any? = null, @Query("parameter2") parameter2: Any? = null): Call<RequestResult>
@Headers(
"Content-Type: application/x-www-form-urlencoded"
)
@POST("/application/x-www-form-urlencoded")
fun postUrlencoded(@QueryMap parameters: Map<String, String>? = null): Call<RequestResult>
@Headers(
"Content-Type: application/json"
)
@GET("/application/json")
fun getJson(@Query("json") json: Map<String, String>? = null): Call<RequestResult>
@Headers(
"Content-Type: application/json"
)
@POST("/application/json")
fun postJson(@Body parameters: Map<String, String>? = null): Call<RequestResult>
}
}
baseUrl은 기본이 되는 URL주소입니다.
- www.example.com/apple
- www.example.com/banana
위와 같은 주소가 있다면 baseUrl은 www.example.com이 됩니다.
addConverterFactory는 Response를 Convert해주는 Factory들을 추가합니다.
GsonConverterFactory는 Gooogle의 Gson을 통해 Json, XML을 파싱할 수 있고, ScalarsConverterFactory는 String으로 파싱할 때 사용합니다.
Interface
Interface로 API를 정희하고 Retrofit을 통해 Interface를 구체화한 객체를 통해 API를 호출할 수 있습니다.
interface RetrofitHTTP {
@Headers(
"Content-Type: application/x-www-form-urlencoded"
)
@GET("/application/x-www-form-urlencoded")
fun getUrlencoded(@Query("parameter1") parameter1: Any? = null, @Query("parameter2") parameter2: Any? = null): Call<RequestResult>
@Headers(
"Content-Type: application/x-www-form-urlencoded"
)
@POST("/application/x-www-form-urlencoded")
fun postUrlencoded(@QueryMap parameters: Map<String, String>? = null): Call<RequestResult>
@Headers(
"Content-Type: application/json"
)
@GET("/application/json")
fun getJson(@Query("json") json: Map<String, String>? = null): Call<RequestResult>
@Headers(
"Content-Type: application/json"
)
@POST("/application/json")
fun postJson(@Body parameters: Map<String, String>? = null): Call<RequestResult>
}
- @Headers : HTTP Header를 설정할 수 있습니다.
- @GET : HTTP Method를 정의합니다. (POST, PUT, DELETE 등 HTTP 표준에서 제공하는 Method를 사용할 수 있다.)
- @Query : Paramter를 설정할 수 있다.
- @QueryMap : 복잡한 Paramter를 Map으로 설정할 수 있다.
- @Body : HTTP Body를 설정할 수 있다.
그 외의 Annotation(https://square.github.io/retrofit/)
동기처리
execute()를 통해 Response를 받는다.
thread {
val response = RetrofitBuilder.retrofit.getUrlencoded(parameter1, parameter2).execute()
CoroutineScope(Dispatchers.Main).launch {
if (response.isSuccessful) {
binding.resultTextView.text = response.body()?.toString()
} else {
binding.resultTextView.text = response.errorBody()?.toString()
}
}
}
비동기처리
enqueue(callback)를 통해 Callback을 구현한다.
RetrofitBuilder.retrofit.getUrlencoded(parameter1, parameter2).enqueue(object : Callback<RequestResult> {
override fun onResponse(call: Call<RequestResult>, response: Response<RequestResult>) {
if (response.isSuccessful) {
binding.resultTextView.text = response.body()?.toString()
} else {
binding.resultTextView.text = response.errorBody()?.toString()
}
}
override fun onFailure(call: Call<RequestResult>, t: Throwable) {
binding.resultTextView.text = t.toString()
}
})
주의할 점
Status Code가 400번대 300번대 등 실패시에도 onResponse가 실행된다. isSuccessful로 확인해야한다.
Git (예제코드)
github.com/KangTaeJong98/Example/tree/main/Android/HTTP
KangTaeJong98/Example
My Example Code. Contribute to KangTaeJong98/Example development by creating an account on GitHub.
github.com
'Android (안드로이드) > HTTP' 카테고리의 다른 글
Android in A..Z - HTTP (Volley) (0) | 2021.03.16 |
---|---|
Android in A..Z - HTTP (URLConnection) (0) | 2021.03.12 |
Android in A..Z - HTTP (개념) (0) | 2021.03.12 |