Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- Algorithm
- DataBinding
- View
- sqlite
- Android
- Navigation
- Coroutine
- CustomView
- HTTP
- hilt
- 안드로이드
- kotlin
- 알고리즘
- Behavior
- lifecycle
- activity
- 코틀린
- onMeasure
- 백준
- notification
- ViewModel
- 알림
- BOJ
- CoordinatorLayout
- recyclerview
- CollapsingToolbarLayout
- onLayout
- LiveData
- AppBarLayout
- room
Archives
- Today
- Total
개발일지
Android in A..Z - Notification (Action) 본문
Notification Action
알림을 빠르게 대응할 수 있도록 알림을 탭했을 때 작업을 추가 하거나 최대 3개의 Action을 추가할 수 있다.
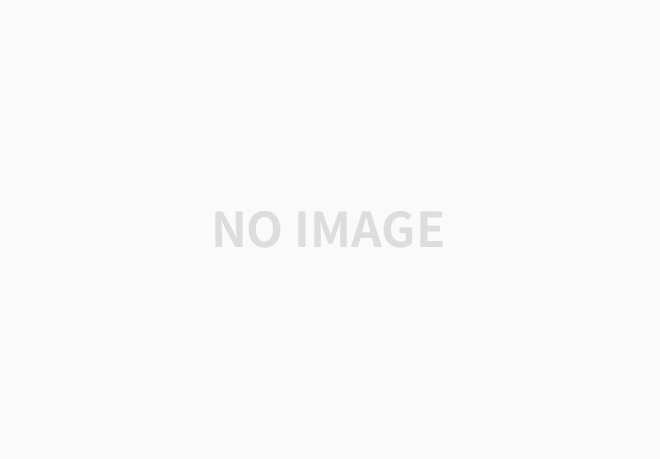
setContentIntent
알림을 탭 했을 때 PendingIntent를 통해 작업을 정할 수 있다.
private fun createPendingIntent(message: Message): PendingIntent {
return NavDeepLinkBuilder(context)
.setGraph(R.navigation.navigation_main)
.setDestination(R.id.actionFragment)
.setArguments(
Bundle().apply {
putSerializable("message", message)
}
)
.createPendingIntent()
}
fun notify(message: Message) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
createNotificationChannel()
}
val pendingIntent = createPendingIntent(message)
val deleteIntent = createDeleteAction(message)
val notification = NotificationCompat.Builder(context, CHANNEL_ID)
.setSmallIcon(R.drawable.ic_android)
.setContentTitle(message.title)
.setContentText(message.message)
.setPriority(NotificationCompat.PRIORITY_MAX)
.setAutoCancel(false) // true면 Notification 클릭시 삭제
.setOngoing(true) // true면 직접 Notification을 지울 수 없음
.setShowWhen(false) // false면 Notification 시간을 보이지 않음
.setContentIntent(pendingIntent) // 알람 클릭시 Intent
.addAction(R.drawable.ic_delete, context.getString(R.string.delete), deleteIntent)
.build()
manager.notify(message.id, notification)
}
addAction
탭 했을 때 작업 외에도 최대 3개의 Action을 추가할 수 있다. addAction도 마찬가지로 PendingIntent를 사용한다.
fun notify(message: Message) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
createNotificationChannel()
}
val pendingIntent = createPendingIntent(message)
val deleteIntent = createDeleteAction(message)
val notification = NotificationCompat.Builder(context, CHANNEL_ID)
.setSmallIcon(R.drawable.ic_android)
.setContentTitle(message.title)
.setContentText(message.message)
.setPriority(NotificationCompat.PRIORITY_MAX)
.setAutoCancel(false) // true면 Notification 클릭시 삭제
.setOngoing(true) // true면 직접 Notification을 지울 수 없음
.setShowWhen(false) // false면 Notification 시간을 보이지 않음
.setContentIntent(pendingIntent) // 알람 클릭시 Intent
.addAction(R.drawable.ic_delete, context.getString(R.string.delete), deleteIntent)
.build()
manager.notify(message.id, notification)
}
코드
@Singleton
class ActionNotificationManager @Inject constructor(
@ApplicationContext
private val context: Context
) {
companion object {
private const val CHANNEL_ID = "com.taetae98.notification.ACTION"
private const val CHANNEL_NAME = "Action"
private const val CHANNEL_DESCRIPTION = "This is Action Notification"
}
private val manager by lazy { context.getSystemService(NotificationManager::class.java) }
fun notify(message: Message) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
createNotificationChannel()
}
val pendingIntent = createPendingIntent(message)
val deleteIntent = createDeleteAction(message)
val notification = NotificationCompat.Builder(context, CHANNEL_ID)
.setSmallIcon(R.drawable.ic_android)
.setContentTitle(message.title)
.setContentText(message.message)
.setPriority(NotificationCompat.PRIORITY_MAX)
.setAutoCancel(false) // true면 Notification 클릭시 삭제
.setOngoing(true) // true면 직접 Notification을 지울 수 없음
.setShowWhen(false) // false면 Notification 시간을 보이지 않음
.setContentIntent(pendingIntent) // 알람 클릭시 Intent
.addAction(R.drawable.ic_delete, context.getString(R.string.delete), deleteIntent)
.build()
manager.notify(message.id, notification)
}
fun cancel(message: Message) {
manager.cancel(message.id)
}
private fun createPendingIntent(message: Message): PendingIntent {
return NavDeepLinkBuilder(context)
.setGraph(R.navigation.navigation_main)
.setDestination(R.id.actionFragment)
.setArguments(
Bundle().apply {
putSerializable("message", message)
}
)
.createPendingIntent()
}
private fun createDeleteAction(message: Message): PendingIntent {
val intent = Intent(context, ActionNotificationReceiver::class.java).apply {
action = ActionNotificationReceiver.ACTION_DELETE
putExtra(ActionNotificationReceiver.MESSAGE, message)
putExtra("id", message.id)
}
return PendingIntent.getBroadcast(context, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT)
}
@RequiresApi(Build.VERSION_CODES.O)
private fun createNotificationChannel() {
val channel = NotificationChannel(CHANNEL_ID, CHANNEL_NAME, NotificationManager.IMPORTANCE_HIGH).apply {
description = CHANNEL_DESCRIPTION
}
manager.createNotificationChannel(channel)
}
}
Git
https://github.com/KangTaeJong98/Example/tree/main/Android/Notification
KangTaeJong98/Example
My Example Code. Contribute to KangTaeJong98/Example development by creating an account on GitHub.
github.com
'Android (안드로이드) > Notification' 카테고리의 다른 글
Android in A..Z - Notification (Custom) (0) | 2021.07.15 |
---|---|
Android in A..Z - Notification (Group) (0) | 2021.07.15 |
Android in A..Z - Notification (Extend) (0) | 2021.07.15 |
Android in A..Z - Notification (기본) (0) | 2021.07.15 |